Class Lane
A lane consists of a number of positions that can either contain a vehicle or be empty (i.e. None
).
Vehicles move into one end (the largest index) and out of the other end (index 0).

Simplifications of reality: A lane consists of a number of discrete positions and all vehicles take up equal space.
What happens to the lane as time increases?
Simplification of reality: A vehicle either stands still or moves one place per time step.
What happens at the ends of Lane
?
The Lane
class does not know what is in front or behind. It is thus "someone else" who makes sure that vehicles enter or leave the lane.
Lane
must provide a method to take out the vehicle on the first position and then return the vehicle that was previously there:

There must also be a method that puts a vehicle to the last place:

If you put a vehicle to the last place and it is not free, you will lose what was on that place:
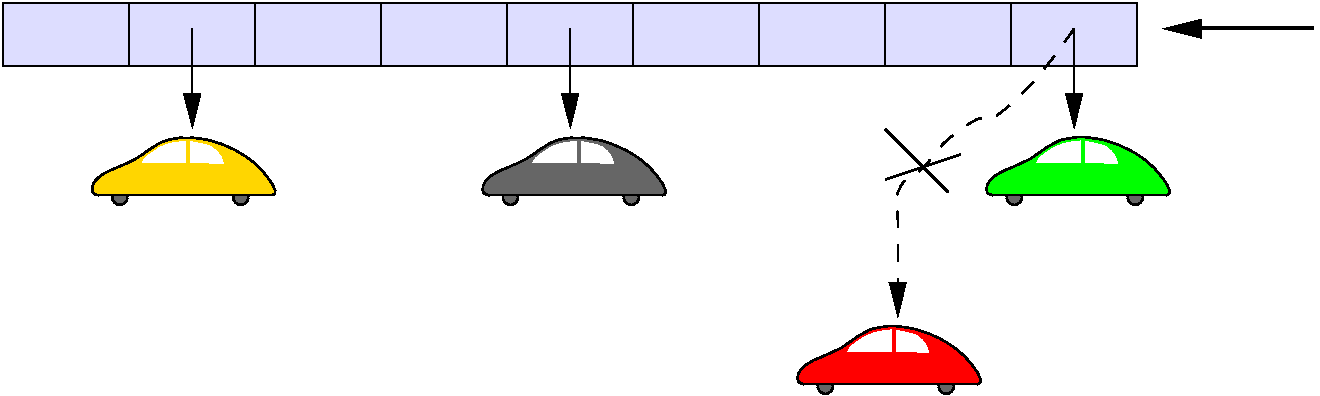
It must also be possible to investigate whether the last place is vacant or not.
The class must include the following methods:
-
A constructor
__init__(length)
that takes the length, i.e. the number of vehicles that can be on the lane. At the beginning there are no vehicles on the lane. -
A method
get_first()
that returns the vehicle at the first position without deleting it. If the first place is empty,None
is returned. -
A method
remove_first()
that returns and deletes the vehicle in the first place. If the place is empty,None
is returned. The method makes no other changes to theLane
object. -
A method
step()
that moves all vehicles except the first one on the file (index 0) one position forward. A vehicle may only be moved if the next position is vacant. The move should take place from left to right, ie. the vehicle on index 1 is moved first, then vehicle on index 2 is moved, and so on. -
A method
is_last_free()
that returnsTrue
if the last place is free, otherwiseFalse
. -
A method
enter(v)
that places vehiclev
at the end of the lane. -
A method
number_in_lane()
that returns the number of vehicles on the lane. -
The method
__str__()
that returns an appropriate string representation of the object. See below!
In the main program, the Lane
objects must be handled with these
methods and thus be independent of the internal representation, i.e. you're not
allowed to access or modify the lane's attribute from outside the object, use
the methods instead!
Below you can find code with print outs that demonstrate the different methods:
Kod | Utskrift |
---|---|
def demo_lane(): """Demonstration of the class Lane""" a_lane = Lane(10) print(a_lane) v = Vehicle('N', 34) a_lane.enter(v) print(a_lane) a_lane.step() print(a_lane) for i in range(20): if i % 2 == 0: u = Vehicle('S', i) a_lane.enter(u) a_lane.step() print(a_lane) if i % 3 == 0: print(' out: ', a_lane.remove_first()) print('Number in lane:', a_lane.number_in_lane()) | [..........] [.........N] [........N.] [.......NS.] out: None [......NS..] [.....NS.S.] [....NS.S..] out: None [...NS.S.S.] [..NS.S.S..] [.NS.S.S.S.] out: None [NS.S.S.S..] [NSS.S.S.S.] [NSSS.S.S..] out: Vehicle(N, 34) [SSS.S.S.S.] [SSSS.S.S..] [SSSSS.S.S.] out: Vehicle(S, 0) [SSSS.S.S..] [SSSSS.S.S.] [SSSSSS.S..] out: Vehicle(S, 2) [SSSSS.S.S.] [SSSSSS.S..] [SSSSSSS.S.] out: Vehicle(S, 4) [SSSSSS.S..] Number in lane: 7 |